Since Swift 4, the recommended way to parse JSON data is to use the Codable
protocols with the JSONEncoder
and JSONDecoder
classes.
However, this method requires creating Swift model types that match the JSON data structure you need to decode.
Sometimes, you might want to avoid creating extra Swift types if you only need to read some of the information in the JSON data locally.
- This article is part of the Parsing JSON in Swift series.
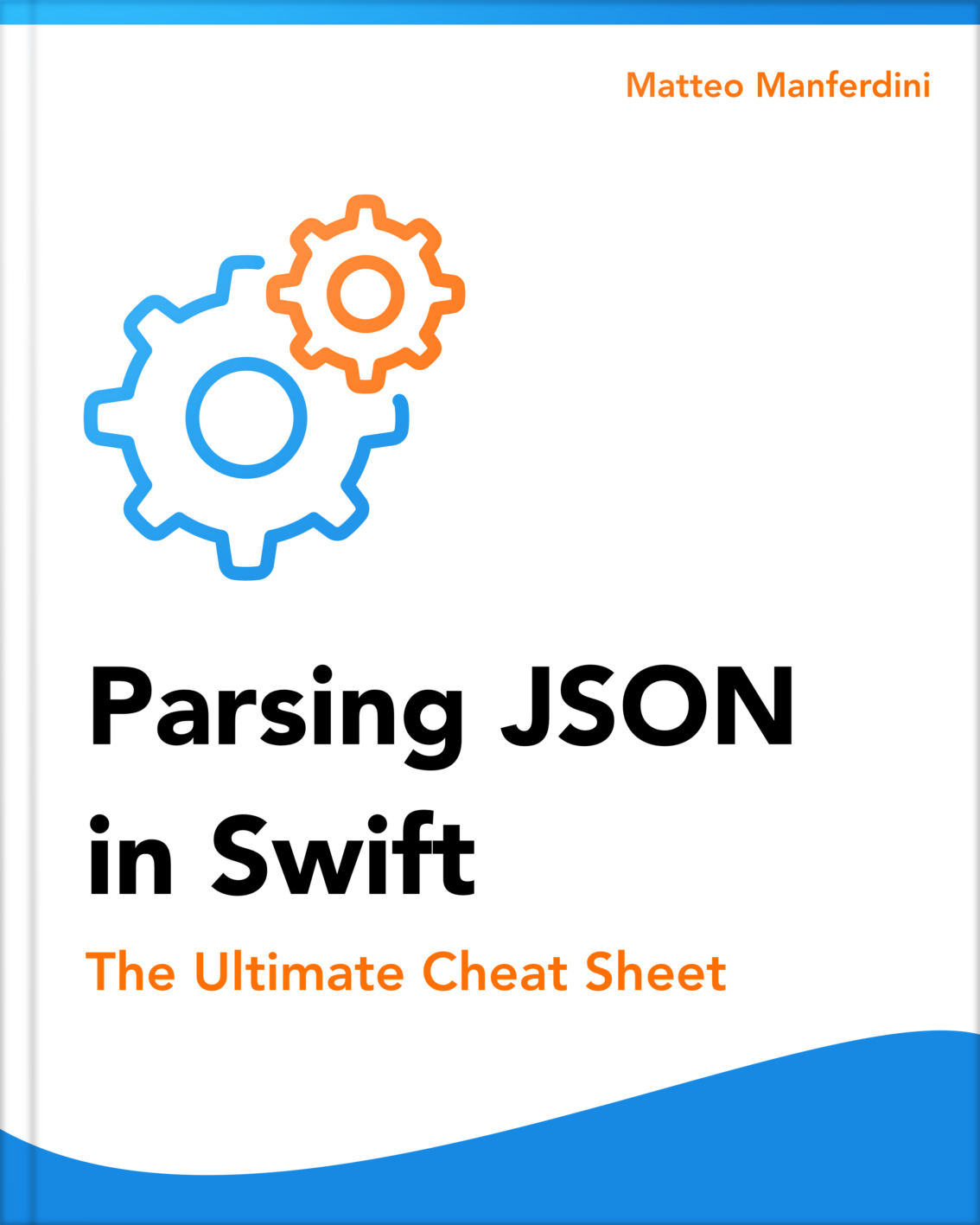
FREE GUIDE: Parsing JSON in Swift - The Ultimate Cheat Sheet
Learn the fundamentals of JSON parsing in Swift, advanced decoding techniques, and tasks for app development
DOWNLOAD THE FREE GUIDEThe Foundation framework contains an old class called JSONSerialization, which was the standard way to decode JSON data before the Codable
protocols were introduced.
The JSONSerialization
class does not require custom Swift types to decode JSON data. Instead, it converts it into dictionaries, arrays, and other standard Swift types.
import Foundation
let data = """
{
"name": "Tomatoes",
"nutrition": {
"calories": 22,
"carbohydrates": 4.8,
"protein": 1.1,
"fat": 0.2
},
"tags": [
"Fruit",
"Vegetable",
"Vegan",
"Gluten free"
]
}
""".data(using: .utf8)!
let json = try JSONSerialization.jsonObject(with: data) as! [String: Any]
let nutrition = json["nutrition"] as! [String: Any]
let carbohydrates = nutrition["carbohydrates"] as! Double
print(carbohydrates)
// Output: 4.8
The disadvantage of this approach is that the content of the resulting dictionaries and arrays is untyped, so you must force-cast any value to the appropriate type.
This produces a lot of boilerplate code, so it should be used sparingly. If the data you decode will be used across your app, you should create Codable
types instead and use a JSONDecoder
instance.
If you want a quick reference of code snippets to parse JSON data in Swift with or without Codable
, together with other JSON parsing techniques, download my free cheat sheet below.
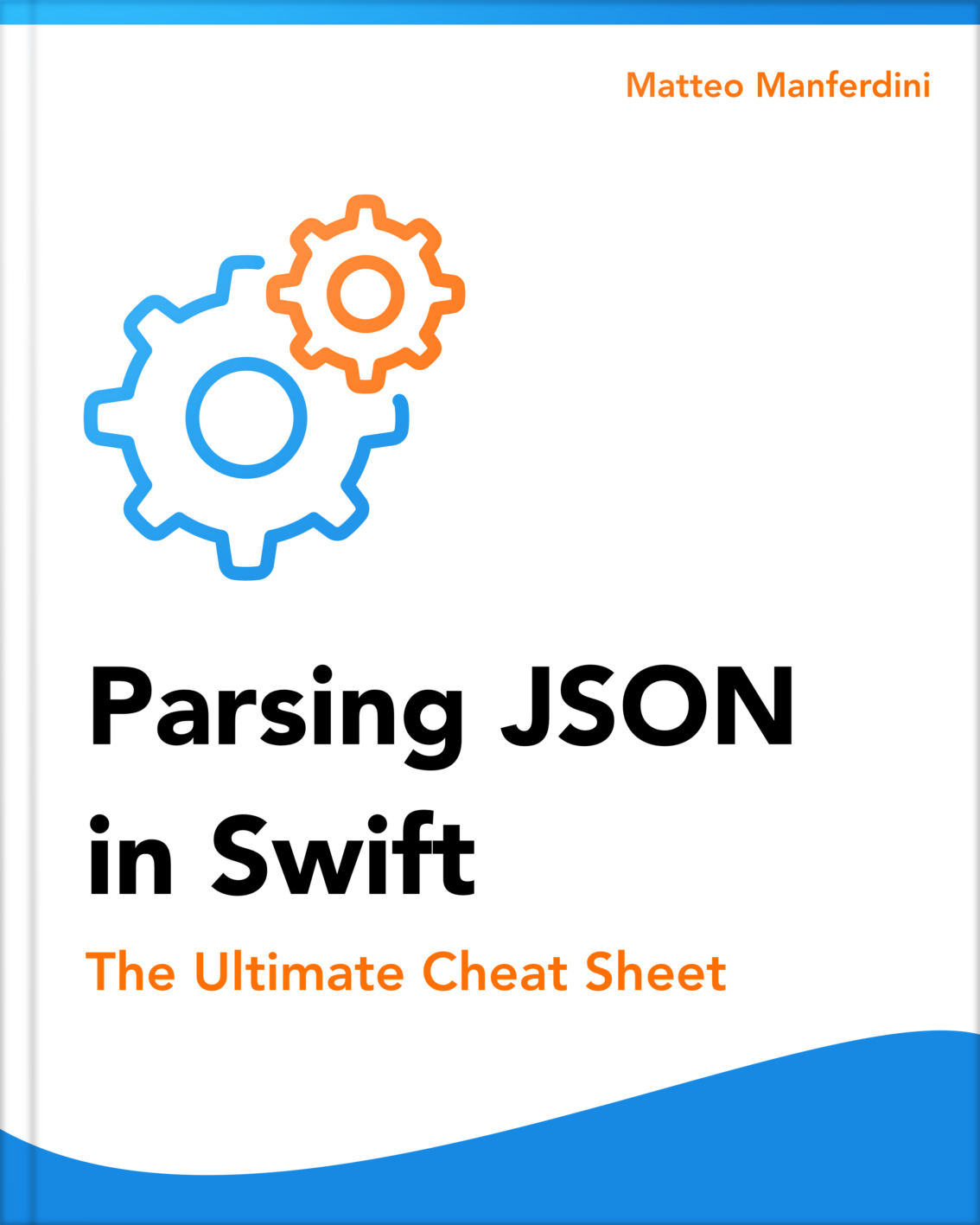
FREE GUIDE: Parsing JSON in Swift - The Ultimate Cheat Sheet
Learn the fundamentals of JSON parsing in Swift, advanced decoding techniques, and tasks for app development
DOWNLOAD THE FREE GUIDEMatteo has been developing apps for iOS since 2008. He has been teaching iOS development best practices to hundreds of students since 2015 and he is the developer of Vulcan, a macOS app to generate SwiftUI code. Before that he was a freelance iOS developer for small and big clients, including TomTom, Squla, Siilo, and Layar. Matteo got a master’s degree in computer science and computational logic at the University of Turin. In his spare time he dances and teaches tango.